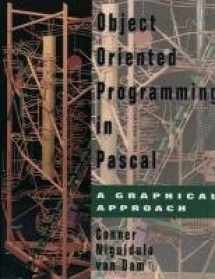
Object-Oriented Programming in Pascal: A Graphical Approach
Book details
Summary
Description
The book you are holding represents a new direction in introductory computer science instruction. For more than a decade, we've been hearing that object-oriented programming OOP is "the wave of the future" and that it has a central place in the computer science curriculum. Recently, the debate has moved from whether OOP belongs in the curriculum to where it belongs. Now, with this book, we present our answer: We think OOP should be taught at the beginning. We don't mean that OOP should be introduced sometime during the first course; OOP should be part of the very first chapter. What's more, the premise of this book is that students need to see all of OOP at the beginning. Students then can write significant object-oriented programs using all of the OOP mechanisms, from inter-object communication via parameters and pointers, to inheritance, virtual methods, and polymorphism, before learning most features of the programming language, such as flow of control, arithmetic, or even base types. Then, as students learn more about the constructs of the language, they can use the tools of object-oriented design to create even more powerful, extensible programs. The power of OOP, we have discovered, can be diluted if students do not see all of the machinery at the beginning, tempting as it might be to delay concepts such as pointers, virtual methods, and polymorphism. It has been our experience that students' programs become too convoluted because they must compensate for missing functionality. Our approach cultivates good object-oriented design habits from the start and puts OOP power to work as early as possible, to allow construction of programs of significant size and complexity. For those of us who have been teaching structured procedural programming, teaching OOP at the start requires a significant shift in mindset. In teaching this new way ourselves, we have occasionally felt uncomfortable giving up "tried and true" pedagogy for this new approach. However, the payoff has been terrific. It's exciting to watch students design and build interactive paint programs less than a month into the course, then create a working arcade game like Tetris just halfway through a semester. It's satisfying to know that students go into later courses with a solid start in object-oriented design and programming. We hope that you too find this approach exciting and worthwhile. Parsing the Title Our title, Object-Oriented Programming in Pascal: A Graphical Approach, may not win any awards for brevity, but we do think it accurately reflects what is truly important and unique about this book. To elaborate, allow us to deconstruct our title. Object-Oriented Programming Object-oriented design and programming form the foundation of this book. In the first eight chapters Part I of the book, we devote our full attention to all the key concepts of OOP: defining objects and instances, sending messages, creating communication links, inheritance, virtual methods, and polymorphism. To make sure these concepts aren't just so many big words, we show how they play out in practice by examining complete Pascal programs. However, these programs do not require learning much Pascal syntax; essentially, all we need are the statements to declare classes, instances, and variables; message passing and parameters; a brief introduction to pointers; and assignment. In the rest of the book, we cover the traditional topics such as arithmetic, flow of control, and arrays, but always from an OOP point of view. Throughout Part I, we also place a heavy emphasis on the design of programs. Knowing how to program is more than learning the syntax of particular language; a good programmer is one who can take a specification and design a solution that will meet the user's needs. An object-oriented approach requires that we think about the program as a system of objects from the beginning of the design process. We emphasize that the objects in the system should be designed for re-use. Although this concept may be a little more abstract, since we are forced to think about more than the task currently at hand, we think it pays off well. In the early chapters, we focus on the use and re-use of objects in existing libraries; later, we spend more time designing new objects that can be used in multiple programs. Learning the technique of "systems thinking" requires practice with systems. Besides the numerous exercises which we will describe in more detail shortly , we also provide examples of programs, where we start with a specification, walk through the design, and show the development of the code. Chapter 8, for example, walks through the development of a simple interactive paint program and ties together the ideas of OOP described in Part I. Of course, we don't leave objects behind once we finish those chapters. Object-oriented design and programming techniques permeate the book. Pascal This text uses Pascal. Specifically, we have written all of the code using Borland Pascal for Windows. Borland provides extensive object libraries with all of its Pascal products. The Borland Pascal for Windows environment is quite friendly, with its debugging and help facilities that both novice and expert programmers can appreciate. As an abbreviation, we refer to the Pascal language and the object-oriented extensions as OOPas for Object-Oriented Pascal . Beyond the tools that come with Borland Pascal for Windows, we've also added our own library of objects for Windows called GP for Graphics Package . These additional classes are not meant as a substitute for the complete package provided by Borland; rather, they are a basic set of graphic user interface GUI tools that provide a way to get started with object-oriented programming. GP has enough functionality that students can use it in advanced courses as well. If you want to run the programs in this book, you need both Borland Pascal for Windows and the GP library. Details on how to obtain GP appear a little later in this Preface. Some of you may be wondering why we didn't use another language; after all, Pascal is not inherently an object-oriented language. We spend a good deal of time examining C++ as well as more "purely" object-oriented languages such as Eiffel and Self. We believe C++ is too large, idiosyncratic, and unsafe for introductory students. It is all too possible for introductory students to get lost in the complexity and obscure run-time errors of C++, when we would rather that they focus on the basics of programming and design. It's been our experience that when we do teach C++ in the fourth course in our computer science sequence , our OOPas-trained students transfer their knowledge quite well. Languages like Eiffel and Self hold a great deal of promise, but, at the moment, are not in common enough use. A Graphical Approach We kept the same subtitle as our earlier work, Pascal on the Macintosh: A Graphical Approach Addison-Wesley, 1987 , for the same reasons. First, interactive graphics programming has become even more crucial for today's computer professionals; the vast majority of programs written on all platforms have graphical user interfaces and the bulk of code in interactive programs lies in their GUIs. Indeed, the reason most of our examples involve various aspects of GUIs is that GUIs are the most common denominator of the types of programs students will write after they complete their studies, and GP gives them a solid foundation in this important area of software. The graphical approach also reinforces our emphasis on objects. To learn how to use objects, students need a library, and it made sense for that library to have a focus. The library could focus on a specific discipline, such as engineering or music, but that would necessarily limit our examples to ideas from that discipline and would assume reader knowledge of that discipline. Rather, we chose graphics as the focus so that very little prior knowledge--essentially, just some basic ideas from high school algebra and geometry--was required for students to understand what the objects are and what they do. We've also found that graphics is particularly attractive for students who are new to programming. It's easy for students to make the connections between objects in their programs and graphical objects that appear on the screen. The graphical user interface is a familiar paradigm for today's students, who are increasingly familiar with computers prior to taking the introductory course. Finally, graphics programs are easier to debug, provide more satisfying results, and are simply more fun than traditional number- and character-crunching programs. The idea of "a graphical approach" also implies that we use graphics to teach programming concepts. You will see illustrations generously distributed throughout the book, especially to explain some of the more notoriously difficult concepts, such as recursion, linked lists, and object interrelationships. FEATURES The Graphics Package GP Library When we began teaching our objects-first approach in 1993, we began with the hypothesis that students could learn OOP from the start only if they were given a set of interesting, building-block objects from which they could create programs. First, however, the students needed access to a set of objects that were powerful enough to build applications, yet could be understood, as we said earlier, with little background in any particular domain. The result is the Graphics Package GP library. Parts of GP are explained in detail in the text; Appendix D also contains descriptions of the GP classes students will need to use. Rather than just read about GP, however, students can explore how it works by loading the library into Borland Pascal. With this software, students have access to basic graphics tools such as lines, colors, and ovals and graphical user interface widgets such as buttons and sliders . In addition, GP contains tools for creating simple animations. The software and the accompanying documentation are being distributed, free of charge, on the Internet. The software distribution site also contains all of the code for the examples in the book, so students can, for example, actually use the paint program described in Chapter 8 and see how GP and OOPas work. To access the software from the Internet, use telnet or ftp to access aw.com. At the prompt, log in as anonymous and use your Internet address as the password. From there, you can change to the [A HREF="ftp://ftp.aw.com/cseng/authors/conner/oopascal/" target=_new>ftp.aw.com/cseng/authors/conner/oopascal directory with the command cd conner.csl.oop. The readme.txt file in that directory contains more detailed instructions and descriptions of all the files you may want to download. Documentation as well as source code also are available via the World Wide Web at the following URL:http://www.cs.brown.edu/software/gp/Object Diagrams and Syntax BoxesWe've also adopted a convention for showing object classes, instances, and the relationships among them. The object diagrams seen throughout the text are based on the notation developed by James Rumbaugh and colleagues in Object-Oriented Modeling and Design Prentice-Hall, 1991 . These illustrations show not just the contents of each object, but how it works as a part of the program's system. Important object classes, such as classes in GP, are described in their own object boxes . A list of object boxes in the main text appears after the Table of Contents. Each new Pascal concept is summarized in a syntax box that describes the concept's purpose, syntax, and usage. We've found that these boxes are useful references, so you will find a list of syntax boxes after the Table of Contents.Exercises for All StudentsEach chapter concludes with a summary and exercises. We've included three types of exercises: Understanding Concepts, which are short-answer or discussion questions; Coding Problems, which ask the reader to write a section of a program, such as an object definition or a method; and Programming Problems, which are complete programming projects that can be completed using the concepts addressed thus far in the book and the object libraries. The topics of the problems come from many different disciplines and activities, and they should prove interesting to more than just computer science majors.WHAT YOU'LL FIND INSIDEThis book is designed for introductory computer science courses and could be used over one or two semesters, or two or three quarters. We expect that a single semester course could cover at least all of Parts I and II, and the strings and array chapters in Part III. Courses that move at a brisker pace could add the sets and files chapters in Part III and/or the chapters of Part IV. We do not assume that every instructor will cover every chapter. Here, then, is a brief description of how the chapters are organized.Part I: ObjectsAs we mentioned earlier, the first eight chapters focus on the primary concepts of object-oriented programming. All of the programs in this chapter are built using GP, or a special "Starter Unit" of objects assembled from several GP objects. We use the object classes of these libraries as building blocks for our programs, starting in Chapter 2. We do cover familiar Pascal territory: the PROGRAM statement; the mainline; declaring variables, procedures, and functions; and using parameters. In addition, we look at the syntax necessary for implementing object-oriented concepts, such as the declaration of objects in the TYPE section of a program, the use of key words such as CONSTRUCTOR and INHERITED , the declaration of links, associations pointers and of subclasses, and the use of units. These chapters form the foundation for the rest of the book, and we strongly recommend that these chapters be covered in order so that students have all of the requisite machinery.Part II: Syntax for Math and Flow of ControlThe second part covers the fundamentals of standard Pascal. Here, we see the concepts that most books discuss at the beginning: numbers, constants, and flow of control. We also introduce recursion in this section of the book and discuss the common bugs that occur when using flow-of-control statements, as well as strategies and tools to use in debugging. Taking advantage of our approach, we use examples in this section that rely on the graphics primitives of GP. We also have tried to put every concept in the book in the context of object-oriented programming. In this section, we discuss how the object oriented tools described in Part I, such as polymorphism, can implicitly perform some of the same results as code explicitly written with flow-of-control statements such as CASE . The OOP tools also allow us to approach some traditional topics in new ways: For example, we can describe recursion as a specific case of sending messages from one instance to another. This use of recursion is revisited in the chapters on data structures.Part III: Built-in CollectionsThe third part addresses some of the more advanced fundamentals of Pascal. We examine characters and strings and the accompanying string functions. We explore one- and two-dimensional arrays and discuss the way Pascal allows us to work with structures such as sets and files. As an example of using the string functions, we also begin exploring the ideas of grammars and parsing. This may provide introductory students with just a small peek at some of the topics they are likely to encounter in later computer science courses. Some instructors may choose to skip the sections or chapters on parsing, sets, and files, since that material is not needed for subsequent chapters.Part IV: Data StructuresThe final part deals with dynamic storage allocation and the data structures that rely on it. The order of our chapters is a little different from most books: We introduce stacks, queues, and then linked lists, followed by trees. We begin by showing how the stack data structure allows nodes to be added and removed in a particular manner from one end of a collection. Queues extend the idea by implementing the addition and removal of nodes using both ends. Linked lists go even further, allowing for insertion and deletion at any point in the list of nodes. Finally, trees add the ability to place nodes on branches. All of these structures, however, are based on the notion that individual nodes within the list are objects, not just records. For this reason, you may find that our approach to inserting and removing nodes from these structures differs from what one sees in a procedural programming approach. By providing our nodes with the necessary methods to insert and delete, we maintain our OOP approach of cooperating objects and create structures that are more flexible through polymorphism than "traditional" dynamic data structures tend to be. Should you have time, the final chapter provides a brief introduction to the analysis of algorithms, as a bridge to upper-level courses.FORMATTING AND NOTATIONSA number of notations are used throughout the text. Code, whether in the text body or separated in the text, appears in Courier. New ideas initially appear in italics. Metavariables in code appear in italicized Courier . Class boxes are summaries of the classes used in the book. Each class box has two or three parts. The first part is the class's title, which also includes a brief description or the class's intended purpose. This is followed by a section describing the class's methods. This section does not necessarily list all of the methods available--just the ones relevant for the part of the book the class is being used in . Consult Appendix D for complete GP documentation. Finally, some class boxes will include a third section describing the class's instance variables. Most class boxes do not include this section, as it is not necessary to know the class's implementation in order to use it effectively. Syntax boxes are summaries of Pascal instructions, and most of these boxes follow the same format. The Purpose section provides a brief overview of when the instruction is used. The Syntax section provides an example of the syntax itself. Parts of the syntax that are programmer-specified as opposed to keywords are enclosed in angle brackets and italicized. A section titled Where describes any limitations on the elements used in the syntax. The Returns section describes the values that a function may return. Finally, the Usage section describes the semantics of the syntax shown. You will also see that syntax boxes appear with a gray background.AcknowledgmentsThis project would not have been worthwhile--or even possible--without the input of students in our introductory class at Brown. Since 1992, students in Computer Science 15 and its predecessor, Computer Science 11 have provided valuable feedback on the book, on GP, and on our entire approach to object-oriented programming and design. We thank them for their patience as we experimented on them and for their many constructive contributions. Each year, a group of students become even more involved with the course as undergraduate teaching assistants. They truly are the engine that makes the course run. The TAs in 1992, 1993, and 1994 particularly helped a great deal with the preparation of this manuscript, from formatting earlier drafts to being the first respondents to the text. We'd like to acknowledge our appreciation for all of the TAs' help, and we'd like to particularly mention Dave Wadhwani, Aris Kavour, and Ed Bielawa, for countless summer hours spent over a hot word processor, and Gwen Shipley, Bidemi Carrol, Ron Palmon, and Ely Greenfield, for taking primary responsibility for helping to get the first drafts of the manuscript into a form that students could use. We also want to thank two individuals who have gone far and above the call of duty. Robert Duvall has been of great assistance in our own transition from a procedural programming to an OOP approach in our class, being involved in discussions ranging from the choice of a language to the creation of assignments. He was deeply involved in developing the first version of GP with Brook Conner, Ed Bielawa, and Ralph Ruiz and has been very helpful in teaching the rest of our TAs to think "the OOP way." Ralph Ruiz has been a crucial part of this book in each of its versions, from being a teaching assistant and then head teaching assistant for the course, to developing the version of GP that uses Windows, to sweating out the figures and code examples throughout the manuscript. Ralph has provided expertise, intelligence, and insight to this collective effort. Our colleagues in the Brown Computer Science Department have encouraged our efforts in developing this introductory course and in designing a curriculum that emphasizes objects throughout our students' careers. Trina Avery, as always, has provided valuable assistance in the editing of this text, and Lori Agresti has done wonders to keep us all in constant communication. Mary-Kim Arnold also provided top-rate copyediting, spending many hours at each phase of the manuscript's development. As a student of the class at the same time, she was especially helpful at finding passages that while grammatically correct were completely baffling to the new student. Addison-Wesley has made an important commitment to this new approach to introductory computer science, and we particularly appreciate the efforts of Lynne Doran Cote, Maite Suarez-Rivas, Peter Gordon, Juliet Silveri, and the editing, production, and marketing staffs. We also want to thank the reviewers who contributed their time and comments to help improve this work: Anthony Baxter University of Kentucky , Kim Bruce Williams College , Leon Levine University of California Los Angeles , Don Retzlaff University of North Texas , Janice T. Searleman Clarkson University , and Allen Tucker Bowdoin College , Pat Lewis provided valuable comments. Our families have been most supportive throughout the development of this project, showing much patience, understanding, and affection, even when we fell into our "writing moods." Our warmest thank you's for tolerating us go to Mary-Kim, to Reina and Gregory and Gregory's new brother or sister , to Debbie, and to all of our families and friends. We hope you will provide your thoughts and reactions to this book and its approach; you can contact us via e-mail atdbc@cs.brown.edu Brook Conner ,dan@cs.brown.edu David Niguidula , andavd@cs.brown.edu Andy van Dam . Enjoy! Providence, Rhode Island D.B.C.D.A.M.A.v.D.020162883XP04062001
We would LOVE it if you could help us and other readers by reviewing the book